Signum Plotting & Mining Technical Information
Technical Information
public static long getBlockReward(int height) {
if (height == 0 || height >= 1944000) {
return 0;
}
int month = height / 10800;
return
BigInteger.valueOf(10000).multiply(BigInteger.valueOf(95).pow(month))
.divide(BigInteger.valueOf(100).pow(month)).longValue() * Constants.ONE_BURST;
}
The Process of Mining and Forging Blocks
A Signum Node (locally installed or accessed remotely) and Signum mining software (to compute deadlines from plot files) are required.
The mining software requests mining information from the node as follows:
- new generation signature = Shabal-256 (previous generation signature, previous block generator)
- base target value
- new block height
- generation hash = Shabal-256 (new generation signature, new block height)
From this information (API Example), the mining software produces the following:
- scoop number = modulo 4096 (generation hash)
This number is used to select scoops (scoop data) from the plot files. For each scoop, the following are calculated:
- target = shabal-256 (scoop data, generation signature)
- deadline = 1st 8 bytes (target / base target)
After all of the deadlines have been calculated, the lowest deadline (subject to the maximum deadline setting), if any, is submitted to the node, along with the numeric account ID that is bound to the related plot file, and the nonce number for the scoop data that was used to generate the deadline. For solo mining, the passphrase of the account bound to the plot file is also passed. For pool mining, the passphrase of the pool account is used.
Note: Older mining software submitted multiple, subsequently lower deadlines as they were found, subject to the maximum deadline setting. However, there is no reason to do this following the Sodium hardfork.
Upon receiving the deadline (or deadlines) from the mining software, the receiving node creates a nonce that will be used to find and verify the deadline. If the deadline is verified, the node waits for the deadline to expire. If a lower deadline is passed to the node while the original deadline is expiring, the node will wait for the new lower deadline to expire. After the lowest deadline submitted to the node has expired, the node will check the network to see if a new valid block has already been announced. If a new block has already been announced, the information will be discarded. If a new block has not been announced, the node will begin forging a new block.
To forge a block, the node collects unconfirmed transactions and checks the validity of each transaction, signature, timestamp, etc. It assembles as many transactions as possible until the maximum number of transactions per block is reached or all available transactions have been processed. The constraints on including transactions are the maximum block payload of 179,520 bytes (176 kB) and the maximum number of transactions includable in a single block. The theoretical maximum number of transactions is 19,200.
See Signum transaction processing for more information on how transaction processing works.
Once a node forges a block, it announces it to the network by connecting to peers and sending the block for verification and validation.
Note: Transactions are stored separately from blocks.
Pools often set a maximum deadline. Deadlines exceeding this limit are excluded when calculating historical shares.
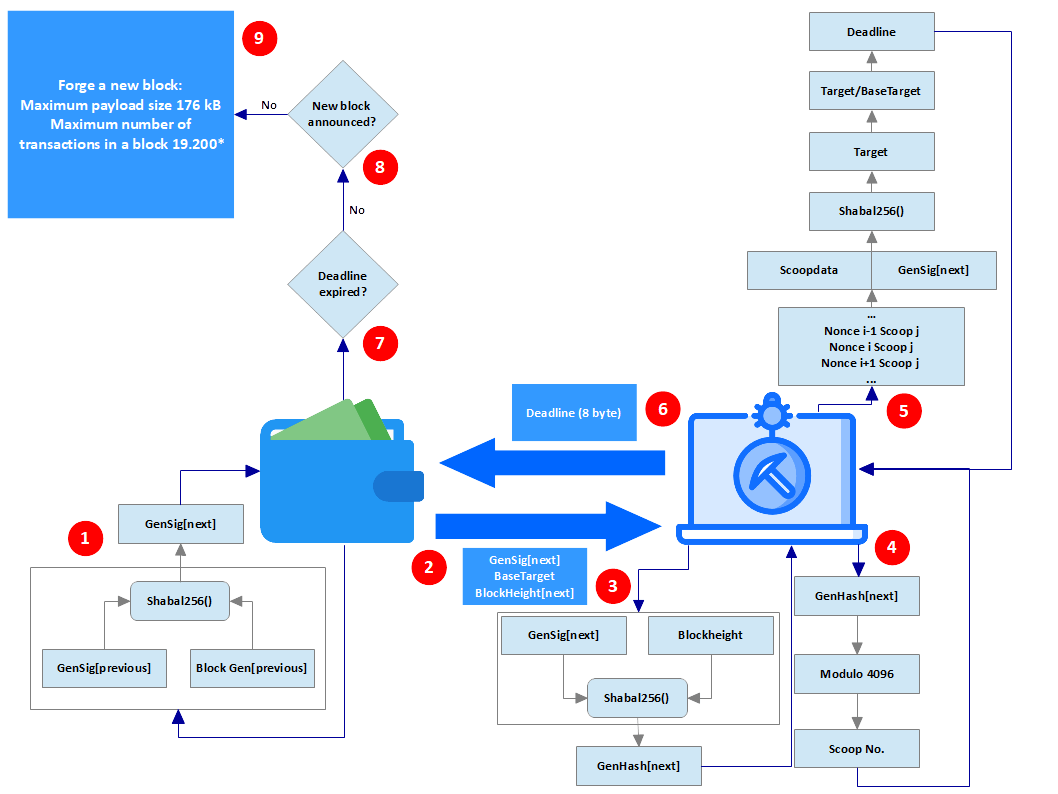
Block Contents and Block Explorers
Signum block explorers are used to view block information and contents. Block explorers are provided by programmers and organizations within the Signum community. Various block explorers can be found in the online services directory. A selection of details for each block is also included in most wallets.
Information typically found in a block explorer:
- Block version number – refers to the block format which determines what a block can contain.
- Block height
- List of included transaction Ids.
- Payload hash – Sha256 hash of all the data included in the block payload.
- Timestamp – time the block was forged – derived from the time of the Genesis block (August 11th, 2014, at 02:00:00)
- Total amount of all included transactions
- Total amount of transaction fees
- Payload length
- The public key of the account that forged the block.
- Generation signature used to forge the block.
- Sha256 hash of the previous block’s contents.
- Previous block Id – first 8 bytes of the previous block hash converted to a number.
- Cumulative difficulty – used to prevent “Nothing at Stake” problems during potential forks: Calculation: (previous cumulative difficulty + ( 18446744073709551616 / base target )
- Base target that was used when the block was forged.
- Nonce number used to forge the block.
- AT – payload bytes of the AT if AT was added to the block.
- Block signature – 64-byte hash generated from the forger’s private key and the block’s contents.
Technical Information for Creating Plot Files
Code (Java): https://github.com/signum-network/signumj/blob/master/src/main/java/signumj/crypto/plot/impl/MiningPlot.java
Following is the terminology necessary for understanding the plot file creation process in Signum mining:
- Account ID: The Signum account numeric ID that binds a plot file to a specific Signum account.
- Shabal-256: The principle cryptographic function used for Signum processes.
- Seed: A Shabal-256 argument. It can be considered an input variable.
- Hash: In the context of Signum, the output of the Shabal-256 function. Size on disk: 32-Byte (256-bit). All hashes are stored with a final hash.
- Scoop: Scoops are the base level subdivisions of hash data in a plot file. Each scoop contains two hashes. Each scoop is assigned a unique number ranging from 0 – 4096. Size on disk: 64 bytes.
- Nonce: Nonces are the top-level subdivision of hash data in a plot file. Each nonce contains 4096 scoops. Each nonce is assigned a unique number ranging from 0 to (( 2 ^ 64) – 1) (0, 1, 2, 3 … 18,446,744,073,709,551,615). The identifying number is pre-assigned and used as a seed in the nonce’s generation. Because of this, each nonce has a unique set of data. Size on Disk: 256 Kilobytes.
- Plot file: A computer file containing all data necessary for forging Signum blocks. Plot file data is first subdivided by nonces and then by scoops. Size on Disk: minimum of 256 Kilobytes, maximum of total disk capacity.
Note: Plot files contain only raw data. There are no headers. The filename includes all of the information needed by a user or miner. The formatting of the filename is as follows.
POC2 format: AccountID_StartingNonce_NrOfNonces
Generating a Nonce
Step 1: Take the account ID and nonce number you want to plot, encode the account ID as a big-endian unsigned long (64 bit), encode the nonce number as an unsigned long (64 bit). The encoded ID is the first part of the seed, the nonce number is the second. This gives a 16 byte / 128 bit seed.
Hash #8191 = Shabal256 ( 16 byte seed )
Each subsequent hash number will decrease by 1 until the final hash number is 0.
Step 2: Prepend hash #8191 to the initial seed, creating a new seed. Calculate hash #8190.
Hash#8190 = Shabal256 ( Hash#8191, 16 byte seed )
Step 3: Prepend hash #8190 to hash #8191, creating the next seed. Calculate hash #8189.
Hash#8189 = Shabal256 (Hash#8190, Hash#8191, 16 byte seed )
Step 4: Continue prepending each result to the last seed and running the calculation until completing 128 iterations.
The resulting seeds will exceed 4,096 bytes. For all remaining iterations, use only the last 4,096 bytes.
Step 5: Calculate a final Shabal-256 hash of all 8,192 hashes and the original 16-byte seed.
Final Hash = Shabal256( Hash#0, Hash#1, Hash#2, ( . . . ) , Hash#8191, 16 byte seed
Reference Files: (Large files, please see the preview below before downloading.)
Use the Final Hash to XOR All Other Hashes Individually
The XOR logical operator compares the 1st byte from each hash and outputs a ‘1’ if the bytes match or a ‘0’ if the bytes do not match. The operation is performed for each byte position.
Hash 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Hash 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 |
XOR | 1 | 1 | 1 | 1 | 1 | 1 | 1 | 0 |
Hash 1 | 0 | 0 | 0 | 0 | 1 | 1 | 1 | 1 |
Hash 2 | 0 | 0 | 0 | 0 | 1 | 1 | 1 | 0 |
XOR | 1 | 1 | 1 | 1 | 0 | 0 | 0 | 1 |
As follows:
32byte Hash #8191 | XOR | 32byte Final Hash |
32byte Hash #8190 | XOR | 32byte Final Hash |
32byte Hash #8189 | XOR | 32byte Final Hash |
( . . . ) | ||
32byte Hash #0 | XOR | 32byte Final Hash |
When complete, the newly created nonce is stored in a plot file, and the process for generating a nonce repeats. Each subsequent nonce generated is added to the plot file. The number of nonces includable in a plot file is limited only by storage capacity.
POC2 Format
The process for creating nonces described until this point encapsulates what is known as the POC1 format. To address a largely theoretical “time-memory tradeoff” vulnerability with POC1, POC2 was created. Creating the POC2 follows the POC1 format, but a final step is added to reorganize the data. The nonce is divided into 2 halves (scoop numbers 0 – 2047 and scoop numbers 2048 – 4095). The data in the 2nd half of each scoop in the lower numbers and the data in the 1st half of each scoop in the higher numbers are swapped. The mirror scoop is calculated as 4095 – CurrentScoop.
Note: Poc1 plots can till be used for mining, however, the reading speed of POC2 files is half the reading speed of POC1 files. POC1 files can be upgraded to POC2 using a plot file converter. The conversion process takes about as long as replotting, so most opt to replot rather than use the conversion process. You can identify POC1 plot files by their filename, which follows the pattern of 15286677094439976801_64658021_19075408_19075408 (4 numbers separated by underscores). POC2 plot files have only 3 numbers in their file name as follows: 15286677094439976801_1816799207619978854_456192.
Plot Structure
Mining software reads from one or more plot files. It opens a file, locates a scoop, and reads the data that the scoop contains. If the plot file is not optimized for this process, the scoop locations will be in more than one location. In the following example, the software is looking for scoop #403. The continuity is interrupted as the software must read scoop #403 from nonce 0 and then locate and read scoop #403 from nonce 1. Optimization is the process that places all of the scoops from all of the nonces into one sector.
Recently developed software automatically optimizes plot files as they are written to storage. Before this development, it was necessary to use a second program to regroup the data remedially.
Information Deprecated with POC2 Format
Stagger – A group of nonces in a plot file. Each stagger has a stagger number equal to the number of nonces in the group. To find the number of groups in a plot file, the number of nonces is divided by the stagger number. If the stagger number is equal to the number of nonces in the file, there is only one group, and the plot file is completely optimized. If the division does not result in an integer, the plot file can be assumed to be broken. Files names under the POC1 format are as follows:
POC1 format: AccountID_StartingNonce_NrOfNonces_Stagger (deprecated)
Block Reward Schedule
Month | Date | Height | Reward |
---|---|---|---|
0 | 2014-08-11 | 0 | 10000 |
1 | 2014-09-11 | 10800 | 9500 |
2 | 2014-10-11 | 21600 | 9025 |
3 | 2014-11-11 | 32400 | 8573 |
4 | 2014-12-11 | 43200 | 8145 |
5 | 2015-01-11 | 54000 | 7737 |
6 | 2015-02-11 | 64800 | 7350 |
7 | 2015-03-11 | 75600 | 6983 |
( … ) | ( … ) | ( … ) | ( … ) |
83 | 2021-07-11 | 896400 | 141 |
84 | 2021-08-11 | 907200 | 134 |
85 | 2021-09-11 | 918000 | 127 |
86 | 2021-10-11 | 928800 | 121 |
87 | 2021-11-11 | 939600 | 115 |
88 | 2021-12-11 | 950400 | 109 |
89 | 2022-01-11 | 961200 | 104 |
90 | 2022-02-11 | 972000 * | 100 |
*The minimum block reward is 100 Signa for all blocks after block height 972000.
Credits
Information in this documentation is based on an article written by Quibus. The documentation has been revised by decrescendo. Latest revision 7/14/2022. Content auditing for this document is appreciated.